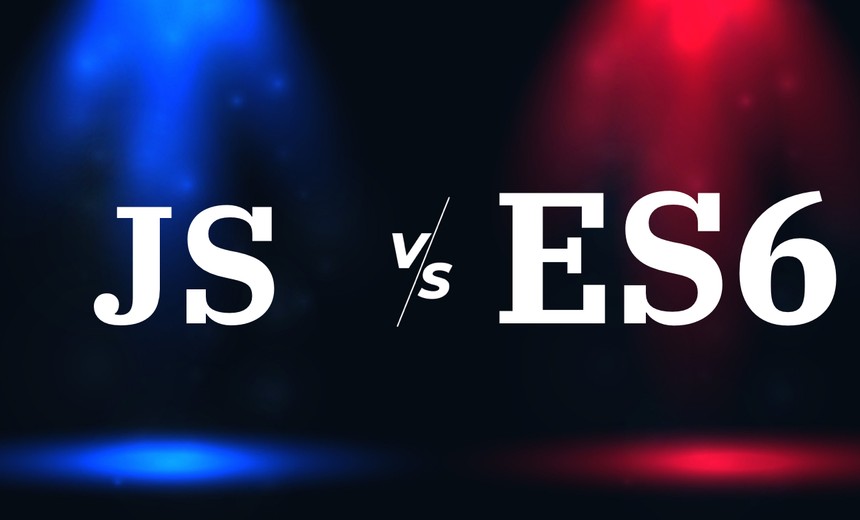
In this blog, we will discuss some strategies of new ES6 syntax for write less and do more.
Boolean Casting
Recommended method according to airbnb’s style guide.
❌ Old Way:
const age = boolean(input.value)
✅ New Way
const age = !!input.value
Optional Chaining
Reading deeply nested property without the hassle of checking each time of its validity.
❌ Old Way:
let isValid = user => user && user.address && user.address.zipcode
✅ New Way:
let isValid = address => user?.address?.zipcode
Nullish Coalescsing
Return the right-hand when the left-hand operand is null or undefined.
❌ Old Way:
const temp = getProductById(1)
const product = temp !== null && temp !== undefined ? temp : unknown
✅ New Way:
const product = getProductById(1) ?? unknown
Default Params
Intuitive and straight forward default values for function parameter.
❌ Old Way:
const createUser = (name, email ) => {
const user ={
name: name ?? ‘Unknown’,
email
}
}
✅ New Way:
const createUser = (name = ‘Unknown’, email) => {
const user = { email, name }
}
Spread Operator
Merge two object into one using this cool syntax.
❌ Old Way:
const details = { name: ‘Man utd’ }
const stats = { games: 7, points: 21 }
const team = Object.assign(
{},
details,
stats
)
✅ New Way:
const details = { name: ‘Man utd' }
const stats = { games: 7, points: 21 }
const team = {
…details,
…stats
}
Destructuring Object
Write less code by unpacking properties from object into distinct variables.
❌ Old Way:
var obj = { a: 1 }
var a = obj.a
var b = obj.b === undefined ? 2 : obj.b
✅ New Way:
var obj = { a: 1 }
var { a, b = 2 } = obj
String Interpolation (Template Literals)
Template literals are literals delimited with backtick (`) characters, allowing for multi-line strings, for string interpolation with embedded expressions, and for special constructs called tagged templates
❌ Old Way:
let dispatchMessage = ‘Hurray, your order with order id ’ + orderId +
‘has been dispatched successfully’
✅ New Way:
let dispatchMessage = `Hurray, your order with order id ${orderId} has been dispatched successfully`
Leave a comment
To make a comment, please send an e-mail using the button below. Your e-mail address won't be shared and will be deleted from our records after the comment is published. If you don't want your real name to be credited alongside your comment, please specify the name you would like to use. If you would like your name to link to a specific URL, please share that as well. Thank you.
Comment via email