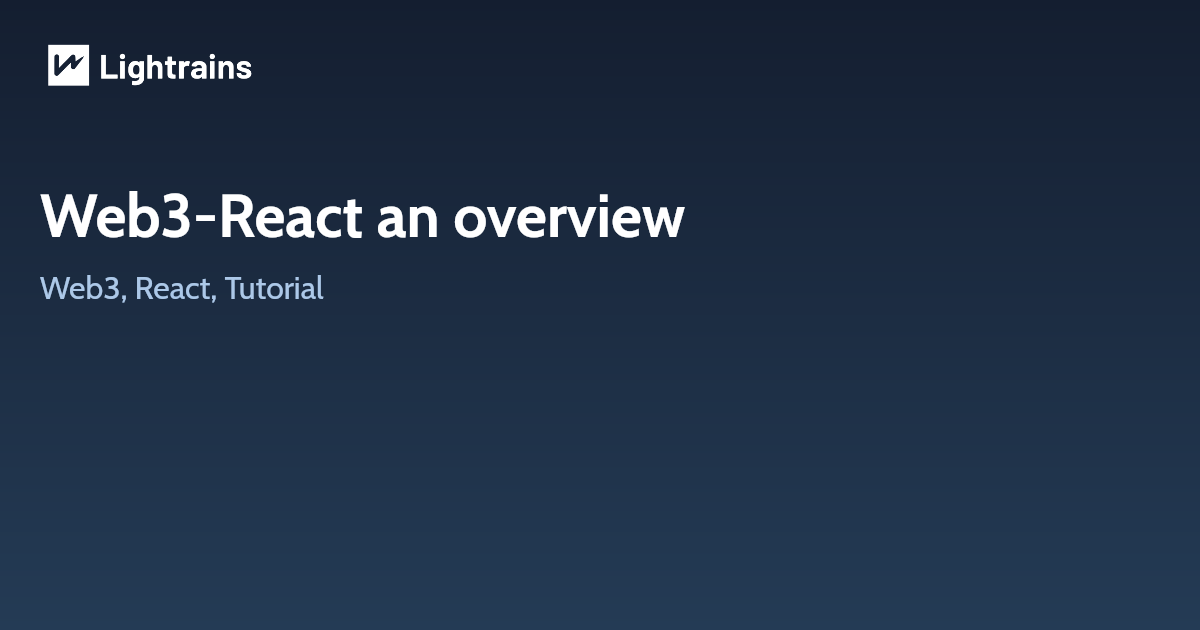
Web3-react is an easy-to-use, simple, extensible web3 framework for building dApps on the Ethereum blockchain network.The ability to create customizable, full-featured connectors that manage every aspect of your dApp’s Ethereum blockchain and user account connectivity is a huge plus point.
It is also a developer-friendly environment that contains an instantiated ethers.js or web3.js instance, the current account and network id, and other information that is available globally across your decentralized apps. Another super important feature you will find with web3-react is the ability to easily connect to nodes on the blockchain.
Installing dependencies.
-
Install web3.js “web3.js is a collection of libraries that allow you to interact with a local or remote ethereum node using HTTP, IPC or WebSocket.”
yarn add web3
-
Install @web3-react This library will enable us to easily integrate our react app with web3 and also avail hooks we can listen to.
yarn add @web3-react/injected-connector
Configure Web3 Connector.
we can get started by configuring the web3 connector.
In the root folder (./src)
, create a wallet folder (./src/wallet)
which will contain configuration for connecting to web3.
Create Connect.js file inside ./src/wallet
folder and paste the content below.
import { InjectedConnector } from '@web3-react/injected-connector'
export const injected = new InjectedConnector({
supportedChainIds: [1, 3, 4, 5, 42]
})
For this next file, here is index.js:
Inside index.js import the injected connector:
import { injected } from '../components/wallet/Connectors'
Create a function inside the Home component and call it connect or whatever you want:
function connect() {}
Set the button to call it on click:
<button
onClick="{connect}"
className="py-2 mt-20 mb-4 text-lg font-bold text-white rounded-lg w-56 bg-blue-600 hover:bg-blue-800"
>
Connect to MetaMask
</button>
In order to connect to wallets, we need to install the core dependency. Do that like so:
yarn add @web3-react/core
yarn add web3
Next, we need to set up the provider. Again, here’s all the code and I’ll explain it piece by piece:
Go to app.js
, import Web3ReactProvider and Web3:
import { Web3ReactProvider } from '@web3-react/core'
import Web3 from 'web3'
Create the getLibrary function:
function getLibrary(provider) {
return new Web3(provider)
}
Wrap the Web3ReactProvider as the top most element and pass getLibrary in as a prop:
<Web3ReactProvider getLibrary={getLibrary}>
<Component {...pageProps} />
</Web3ReactProvider>
Go to index.js again and use the useWeb3React hook to get all the needed values:
const { active, account, library, connector, activate, deactivate } =
useWeb3React()
- active: is a wallet actively connected right now?
- account: the blockchain address that is connected
- library: this is either web3 or ethers, depending what you passed in
- connector: the current connector. So, when we connect it will be the injected connector in this example
- activate: the method to connect to a wallet
- deactivate: the method to disconnect from a wallet
Inside the connect function, use the activate function with the injected connector as an argument. This is what initiates the connection to the user’s MetaMask wallet:
async function connect() {
try {
await activate(injected)
} catch (ex) {
console.log(ex)
}
}
However, at the moment, it would just connect but not show the user is connected visually. To do that, let’s do some simple conditional templating. If the user’s wallet is connected or active, then show their address. Otherwise, not connected:
{
active ? (
<span>
Connected with <b>{account}</b>
</span>
) : (
<span>Not connected</span>
)
}
I’m also going to add a Disconnect button and function:
async function disconnect() {
try {
deactivate()
} catch (ex) {
console.log(ex)
}
}
...
<button
onClick={disconnect}
className="py-2 mt-20 mb-4 text-lg font-bold text-white rounded-lg w-56 bg-blue-600 hover:bg-blue-800"
>
Disconnect
</button>
Users can now connect and disconnect their wallets to this application. After this, all sorts of crazy things can be done like sending tokens or interacting with smart contracts which is what opens up a world of possibilities.
This article originally appeared on lightrains.com
Leave a comment
To make a comment, please send an e-mail using the button below. Your e-mail address won't be shared and will be deleted from our records after the comment is published. If you don't want your real name to be credited alongside your comment, please specify the name you would like to use. If you would like your name to link to a specific URL, please share that as well. Thank you.
Comment via email