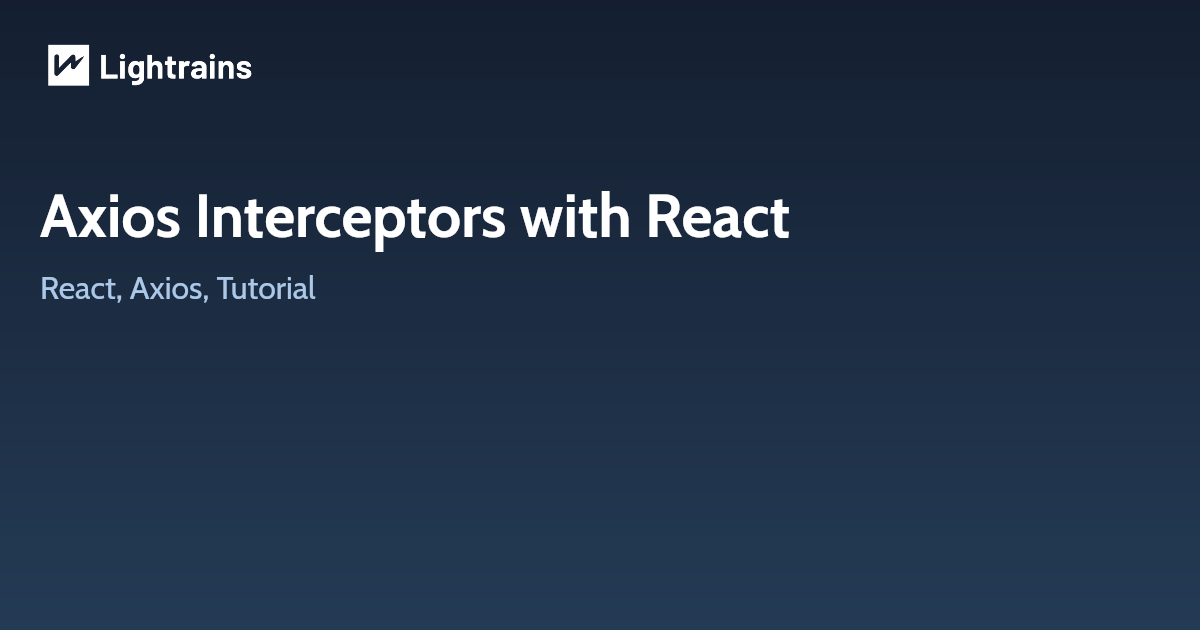
Axios interceptors are the default configurations that are added automatically to every request or response that a user receives. It is useful to check response status code for every response that is being received.
Interceptors are methods which are triggered before or after the main method. There are two types of interceptors:
- request interceptor: - It allows you to write or execute a piece of your code before the request gets sent.
- response interceptor: - It allows you to write or execute a piece of your code before response reaches the calling end.
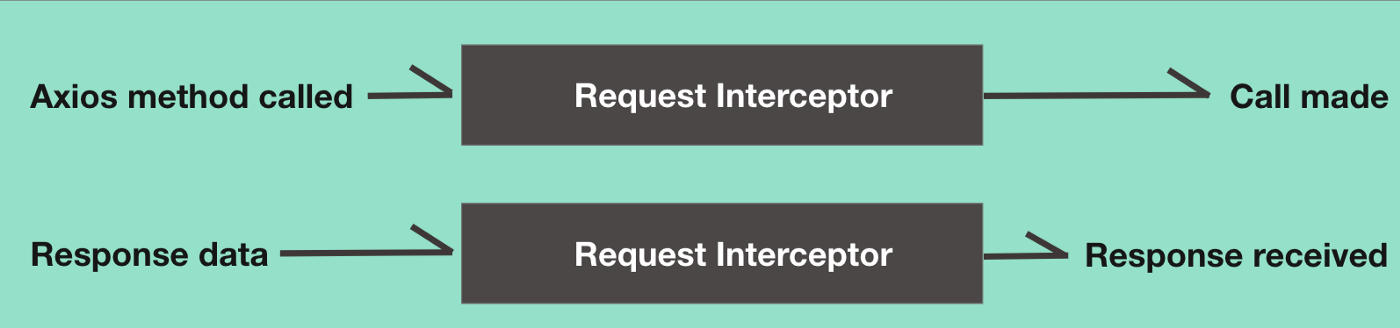
The steps to create Axios request & response interceptors are:
- Create a new Axios instance with a custom config
- Create request, response & error handlers
- Configure/make use of request & response interceptors from Axios
- Export the newly created Axios instance to be used in different locations
Add a request interceptor
import axios from 'axios'
import LocalStorageService from './services/storage/localstorageservice'
import router from './router/router'
// LocalStorageService
const localStorageService = LocalStorageService.getService()
// Add a request interceptor
axios.interceptors.request.use(
config => {
const token = localStorageService.getAccessToken()
if (token) {
config.headers['Authorization'] = 'Bearer ' + token
}
// config.headers['Content-Type'] = 'application/json';
return config
},
error => {
Promise.reject(error)
}
)
Add a response interceptor
axios.interceptors.response.use(
response => {
return response
},
function (error) {
const originalRequest = error.config
if (
error.response.status === 401 &&
originalRequest.url === 'http://127.0.0.1:3000/v1/auth/token'
) {
router.push('/login')
return Promise.reject(error)
}
if (error.response.status === 401 && !originalRequest._retry) {
originalRequest._retry = true
const refreshToken = localStorageService.getRefreshToken()
return axios
.post('/auth/token', {
refresh_token: refreshToken
})
.then(res => {
if (res.status === 201) {
localStorageService.setToken(res.data)
axios.defaults.headers.common['Authorization'] =
'Bearer ' + localStorageService.getAccessToken()
return axios(originalRequest)
}
})
}
return Promise.reject(error)
}
)
Error handling using Axios interceptors
You can use an Axios interceptor to capture all errors and enhance them before reaching your end user. If you use multiple APIs with different error object shapes, you can use an interceptor to transform them into a standard structure.
const axios = require(‘axios’);
axios.interceptors.response.use(
res => res,
err => {
throw new Error(err.response.data.message);
}
)
const err = await axios.get('http://example.com/notfound').
catch(err => err);
// Could not find page /notfound
// err.message;
Conclusion
This is how we can make use of the Axios interceptor functions to intercept every request, update the request with authorization header, API key, etc. if it’s set up like that, and forward the request. Once the request is resolved, take the response, perform predefined checks/filters if any, and return/forward the response to complete the request made.
This article originally appeared on lightrains.com
Leave a comment
To make a comment, please send an e-mail using the button below. Your e-mail address won't be shared and will be deleted from our records after the comment is published. If you don't want your real name to be credited alongside your comment, please specify the name you would like to use. If you would like your name to link to a specific URL, please share that as well. Thank you.
Comment via email