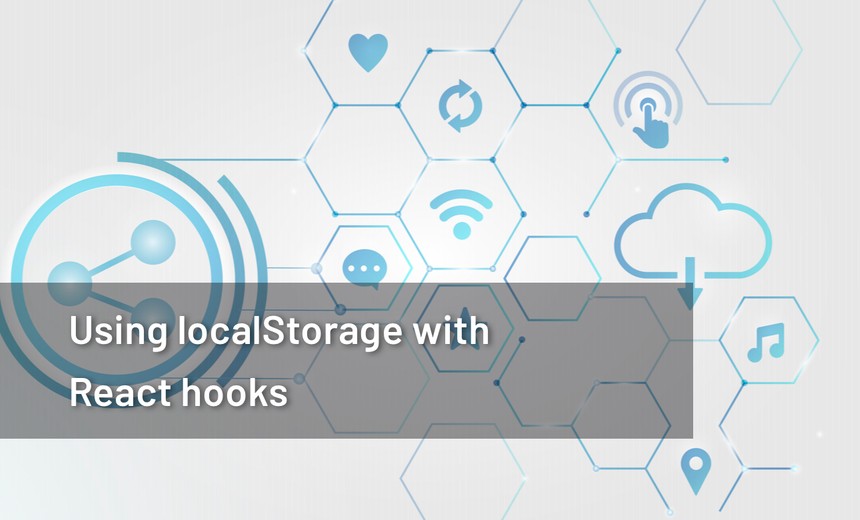
Building more complex JavaScript applications that run in a user’s browser it’s very essential to be able to store information in the browser that is where we can make use of localStorage
Using localStorage with React hooks
In the recent past this would have only been possible with cookies - text files saved to a user’s computer - but the way of managing these with JavaScript was not good. Now there’s a new technology called local storage that does a similar thing, but with an easier-to-use interface.
To save some data, use localStorage.setItem
like this localStorage.setItem('name', 'tom');
useLocalStorage()
Sync state to local storage so that it persists through a page refresh. Usage is similar to useState except we pass in a local storage key so that we can default to that value on page load instead of the specified initial value.LocalStorage is a web storage object to store the data on the user’s computer locally, which means the stored data is saved across browser sessions and the data stored has no expiration time. Local storage can only save strings, so storing objects requires that they be turned into strings using JSON. stringify - you can’t ask local storage to store an object directly because it’ll store “[object Object]”, which isn’t right at all! That also means the object must be run through JSON.
Create the useLocalStorage hook
In a new useLocalStorage.js file, import useState and useEffect from React.
import { useState, useEffect } from 'react'
Create the hook. This hook will accept two arguments, a key and a defaultValue.
const useLocalStorage = (key, defaultValue) => {
// rest of the code?
}
export default useLocalStorage
Inside of the hook, let’s define a state called value . Don’t add any default value for now.
const [value, setValue] = useState()
Inside of the parentheses of the useState , we’ll add a callback function.
const [value, setValue] = useState(() => {})
Inside the brackets of the callback function, we’ll first create a currentValue variable. Then, wrapping everything in a try catch statement, we’ll try to get the item we stored in the local storage of the browser with localStorage.getItem(key) . If the key doesn’t exist, we’ll take the one from the defaultValue we passed as an argument to the hook. Finally, we’re returning the currentValue.
const [value, setValue] = useState(() => {
let currentValue
try {
currentValue = JSON.parse(localStorage.getItem(key) || String(defaultValue))
} catch (error) {
currentValue = defaultValue
}
return currentValue
})
Below the state, we’ll add a useEffect . Inside of it, we’ll set the key we passed to the hook in the local storage of the browser. The value of that key will be the value state.
useEffect(() => {
localStorage.setItem(key, JSON.stringify(value))
}, [value, key])
Finally, we’ll return both the value and the setValue at the bottom of the hook.
return [value, setValue]
Find below the final code for the useLocalStorage hook.
import { useState, useEffect } from 'react'
const useLocalStorage = (key, defaultValue) => {
const [value, setValue] = useState(() => {
let currentValue
try {
currentValue = JSON.parse(
localStorage.getItem(key) || String(defaultValue)
)
} catch (error) {
currentValue = defaultValue
}
return currentValue
})
useEffect(() => {
localStorage.setItem(key, JSON.stringify(value))
}, [value, key])
return [value, setValue]
}
export default useLocalStorage
Import the hook at the top of App.js.
import useLocalStorage from './useLocalStorage'
Call the hook.
const [theme, setTheme] = useLocalStorage('theme', 'dark')
You can use the theme value as well as the setTheme however you want. For example, you can add a button that toggles the theme of the app.
<button
onClick={() => (theme === 'light' ? setTheme('dark') : setTheme('light'))}>
Toggle theme
</button>
How does useLocalStorage works?
- Store a
key/value
pair in the localStorage of the client device. - Sync the stored value with a React state in order to re-render the component when a new value is set.
- Avoiding errors when the hook is invoked at build time or server-side.
Window API: localStorage Can I Use localstorage
Leave a comment
To make a comment, please send an e-mail using the button below. Your e-mail address won't be shared and will be deleted from our records after the comment is published. If you don't want your real name to be credited alongside your comment, please specify the name you would like to use. If you would like your name to link to a specific URL, please share that as well. Thank you.
Comment via email