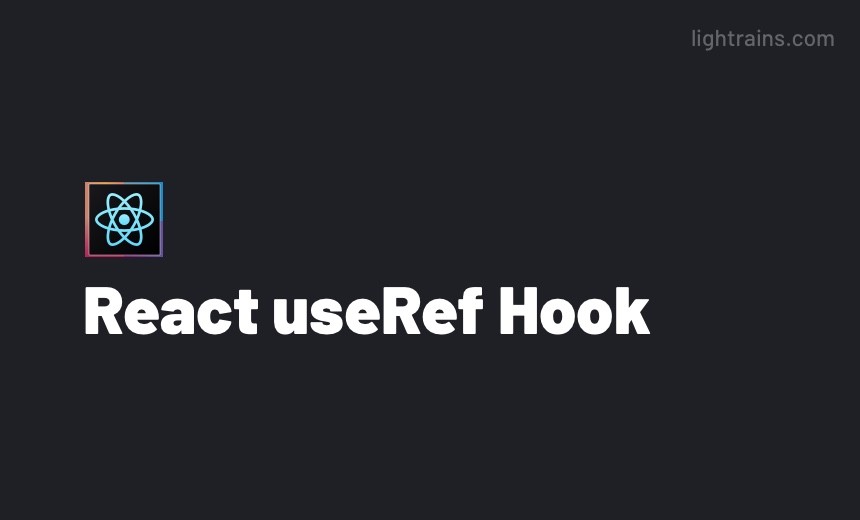
Understanding the React useRef Hook. useRef
is one of the standard hooks provided by React. It will return an object that you can use during the whole lifecycle of the component.
The main use case for the useRef
hook is to access a DOM child directly. I’ll show exactly how to do that in another section.
You can initialize a new ref
inside a component with the following code:
// create a ref
const reference = useRef()
How to use React useRef?
Once created, you can get and set the value of the ref by accessing the .current property of the object, like so:
// create a ref
const reference = useRef()
// set the ref value
reference.current = 'Hello World'
// this prints "Hello World" to the console
console.log(reference.current)
Referencing a value with a ref
Call useRef
at the top level of your component to declare one or more refs
import { useRef } from 'react'
export default function App() {
// create a ref
const counter = useRef(0)
// increase the counter by one
const handleIncreaseCounter = () => {
counter.current = counter.current + 1
}
return (
<div>
<h1>About useRef!</h1>
<h2>Value: {counter.current}</h2>
<button onClick={handleIncreaseCounter}> Increase counter </button>
</div>
)
}
To fix both of these bugs, you should use useState instead of useRef:
import { useEffect, useState } from 'react'
export default function App() {
// create a counter
const [counter, setCounter] = useState(0)
// increase the counter by one
const handleIncreaseCounter = () => {
setCounter(counter + 1)
}
useEffect(() => {
console.log('counter changed to: ', counter)
}, [counter])
return (
<div>
<h1>About useRef!</h1>
<h2>Value: {counter}</h2>
<button onClick={handleIncreaseCounter}> Increase counter </button>
</div>
)
}
The key difference between useState
and useRef
- If you update the state, your component will re-render
- If you update the value stored in your ref, nothing will happen
Difference between Ref and useRef
useRef is used to create a reference object, while ref
is used to access and assign DOM nodes or react components inside the render method to a reference object.
Also, a ref
can be created using the useRef hook or createRef function, which can’t be done the other way.
useRef can be used to reference any type of object, React ref is simply a DOM attribute used to reference DOM elements.
Usage
- Referencing a value with a ref.
- Manipulating the DOM with a ref.
- Avoiding recreating the ref contents.
Leave a comment
To make a comment, please send an e-mail using the button below. Your e-mail address won't be shared and will be deleted from our records after the comment is published. If you don't want your real name to be credited alongside your comment, please specify the name you would like to use. If you would like your name to link to a specific URL, please share that as well. Thank you.
Comment via email