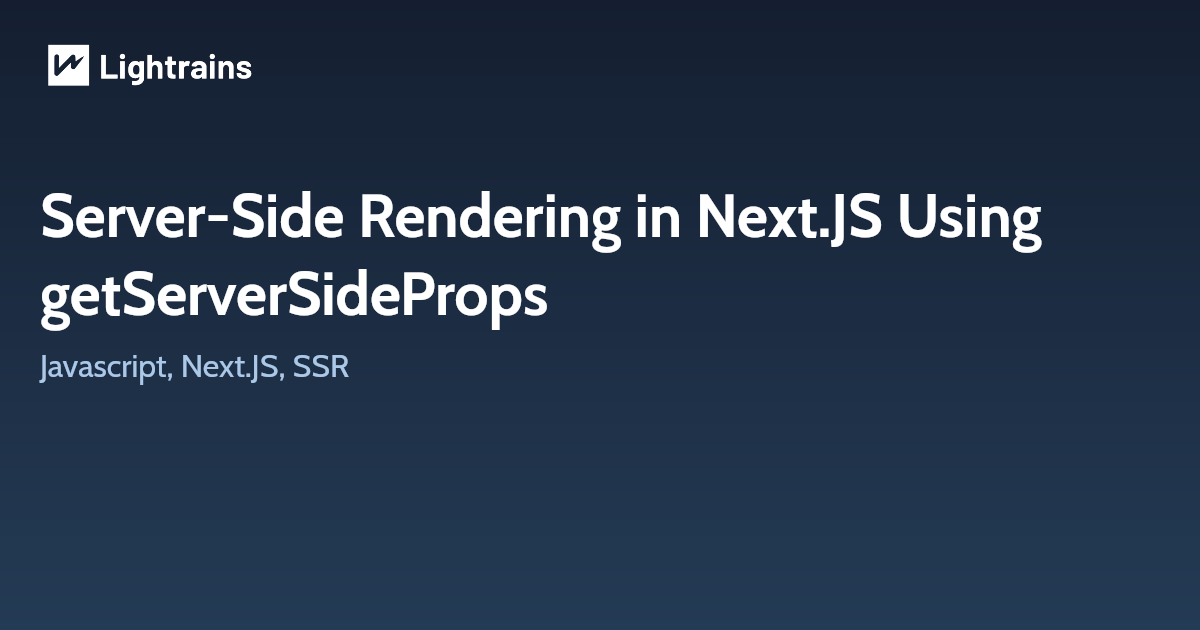
Next.js is an open-source React framework for building production and enterprise-ready applications. It includes many built-in features such as Server-side Rendering (SSR), Static Site Generation (SSG), backend functionality support using API Routes and so on, that make developing web applications a breeze.
What is getServerSideProps?
- This method is primarily used to fetch data for every instance that a user issues a request to the page.
- It fetches the data first before sending the page to the client. Should the client happen to issue a subsequent request, the data is fetched again.
- Using GetServerSideProps allows you to improve your SEO as in this method the data is rendered before it reaches the client.
- As the data is refreshed every time the user loads the page, they can view the updated information at all times.
export async function getServerSideProps(context) {
return {
props: {} // will be passed to the page component as props
}
}
Example
function Page({ message }) {
return (
<>
<h1>Example page</h1>
<h2>{message}</h2>
</>
)
}
export async function getServerSideProps() {
return {
props: {
message: 'Hello world!'
}
}
}
export default Page
What getServerSideProps returns?
-
props An optional serializable object passed to the page component
return { props: { users } }
-
notFound Returns a 404 status and page.
return { notFound: true }
-
redirect An redirect to allow redirecting to internal or external resources. It should match the pattern
{ destination: string, permanent : boolean }
.return { redirect: { destination: '/post', permanent: false } }
What’s the Difference between getStaticProps and getServerSideProps?
getStaticProps
and getServerSideProps
can be defined as follows:
- getStaticProps(): A method that tells the Next component to populate props and render into a static HTML page at build time.
- getServerSideProps(): A method that tells the Next component to populate the props and render into a static HTML page at run time.
Advantages
- Each time a client loads the page, the data is refreshed, ensuring that it is current at the time of their visit.
- Before it reaches the customer, the data is rendered, which is helpful for SEO.
This article originally appeared on lightrains.com
Leave a comment
To make a comment, please send an e-mail using the button below. Your e-mail address won't be shared and will be deleted from our records after the comment is published. If you don't want your real name to be credited alongside your comment, please specify the name you would like to use. If you would like your name to link to a specific URL, please share that as well. Thank you.
Comment via email