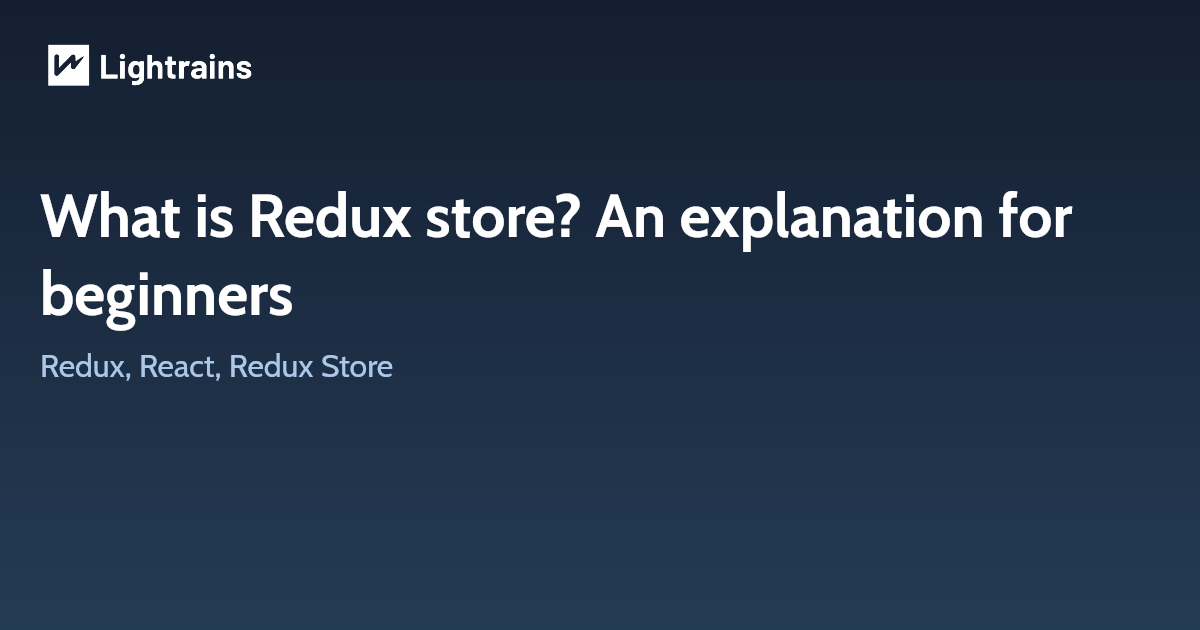
A store is an immutable object tree in Redux. A store is a state container which holds the application’s state. Redux can have only a single store in your application. Whenever a store is created in Redux, you need to specify the reducer. Let us see how we can create a store using the createStore
method from Redux.
A store is not a class
. It’s just an object with a few methods on it. To create it, pass your root reducing function to createStore.
Store Methods
getState()
dispatch(action)
subscribe(listener)
replaceReducer(nextReducer)
getState()
Returns the current state tree of your application. It is equal to the last value returned by the store’s reducer.
dispatch(action)
Dispatches an action. This is the only way to trigger a state change.
The store’s reducing function will be called with the current getState()
result and the given action synchronously. Its return value will be considered the next state. It will be returned from getState()
from now on, and the change listeners will immediately be notified.
subscribe(listener)
Adds a change listener. It will be called any time an action is dispatched, and some part of the state tree may potentially have changed. You may then call getState()
to read the current state tree inside the callback.
replaceReducer(nextReducer)
Replaces the reducer currently used by the store to calculate the state.
It is an advanced API. You might need this if your app implements code splitting, and you want to load some of the reducers dynamically. You might also need this if you implement a hot reloading mechanism for Redux.
The store has several responsibilities:
-
Holds the current application state inside
const store=createStore(reducer)
-
Allows access to the current state via
store.getState() console.log(‘initial state’,store.getState())
-
Allows state to be updated via
store.dispatch(action) store.dispatch(action())
-
Registers listener callbacks via
store.subscribe(listener) store.subscribe(()=>console.log(‘updated state’,store.getState()))
- Handles unregistering of listeners via the unsubscribe function returned by
store.subscribe(listener)
const unsubscribe= store.subscribe(()=>console.log(‘updated state’,store.getState()))
unsubscribe()
Creating a Store
Every Redux store has a single root reducer function. In the previous section, we created a root reducer function using combineReducers
. That root reducer is currently defined in src/reducer.js
in our example app. Let’s import that root reducer and create our first store.
The Redux core library has a createStore API that will create the store. Add a new file called store.js
, and import createStore
and the root reducer. Then, call createStore
and pass in the root reducer:
const store = createStore(rootReducer)
// A simple example
import { createStore } from 'redux'
const BUY_CAKE=‘BUY_CAKE’
function buyCake() {
return {
type:BUY_CAKE,
info:”First redux action”
}
}
const initialState={
numOfCakes=10
}
const reducer=(state=initialState,action)=>{
switch(action.type) {
Case BUY_CAKE:return{
…state,
numOfCakes:state.numOfCakes-1
}
Default:return state
}
}
const store = createStore(reducer)
console.log(‘initial state’,store.getState())
store.dispatch(buyCake())
store.subscribe(()=>console.log(‘updated state’,store.getState()))
export default store
This article originally appeared on lightrains.com
Leave a comment
To make a comment, please send an e-mail using the button below. Your e-mail address won't be shared and will be deleted from our records after the comment is published. If you don't want your real name to be credited alongside your comment, please specify the name you would like to use. If you would like your name to link to a specific URL, please share that as well. Thank you.
Comment via email