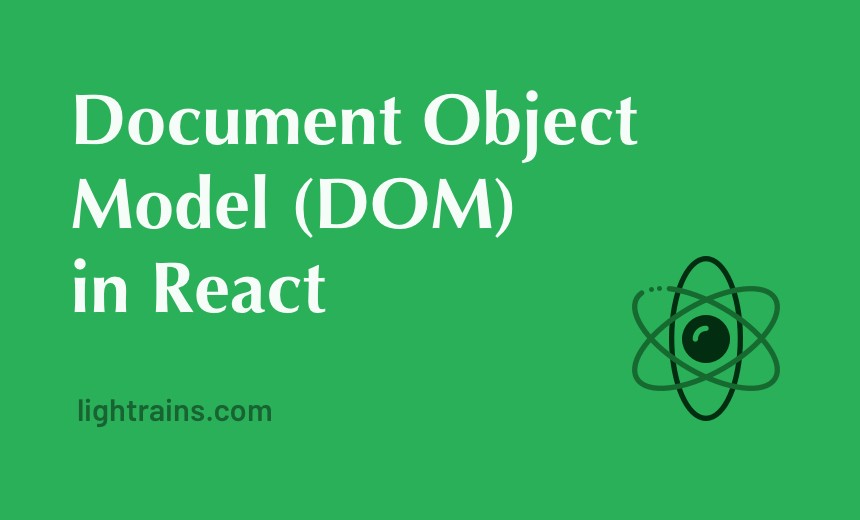
DOM is a blueprint of the webpage. In other words, it represents tree-like structure of elements in a web page. If you are not able to understand what it means, the tree-like structure. Here in this case:
Web page considered as root node, and elements like headings, paragraphs, images and links represents node in the tree.These nodes are connected to each other in a parent-child relationship based on their position in the HTML structure.
For example: Lets say in an HTML document with heading(h1) and two paragrapghs(p) inside a div element. The DOM structure will be:
Document (Root node)
HTML
Body
Div
H1 (heading)
P (paragraph)
p (paragraph)
Each node in the tree can have child nodes, which are elements nested within it, and can also have parent nodes, except for the root node. By representing the web page as a tree-like structure, the DOM enables developers to easily locate, modify, and interact with different elements on the page, making it possible to create dynamic and interactive web applications.
With the DOM, developers can change the content, style, and structure of a web page dynamically. They can add new elements, modify existing ones, and respond to user actions. This allows for creating interactive and dynamic web applications without having to reload the entire page.
The DOM is created when a web page is loaded and rendered by a web browser. These are steps:
- The web browser receives the HTML code of a web page from the server.
- The browser begins parsing the HTML code, interpreting the tags and their attributes.
- As the browser parses the HTML, it constructs the DOM tree by creating nodes for each element encountered.
- The browser continues parsing the HTML, adding child nodes for nested elements and connecting them in a hierarchical structure.
- Once the parsing is complete, the browser has created the full DOM tree, representing the - structure of the web page.
- The browser then uses the DOM to render the web page, displaying the content and applying any associated styles.
Benefits
- Manipulation of web content: Allows dynamically update the content, add or remove elements, change attributes, and apply CSS styles to create dynamic and interactive web applications
- Cross-platform and language independence: Provides a language-independent interface, enabling developers to use various scripting languages like JavaScript, Python, or Ruby to interact with the DOM and manipulate web content.
- Event handling: The DOM provides a mechanism for handling user interactions and events such as mouse clicks, keyboard input, and touch gestures. Developers can attach event listeners to DOM elements to respond to these events and trigger appropriate actions or behaviors in the web application.
- Dynamic updates without page reload: With the DOM, you can update specific parts of a web page dynamically without the need to reload the entire page. This allows for a smoother user experience and faster response times, as only the necessary changes are made to the DOM, resulting in more efficient web applications.
- Accessibility and SEO: The DOM plays a crucial role in making web content accessible to people with disabilities. By ensuring the proper semantic structure of the HTML document and using appropriate techniques, developers can enhance accessibility for screen readers and assistive technologies. Additionally, search engines rely on the DOM to index and understand the content of web pages, affecting search engine optimization (SEO) efforts.
- Integration with other technologies: The DOM seamlessly integrates with other web technologies like CSS and JavaScript libraries/frameworks. CSS styles can be applied and modified through the DOM, while JavaScript libraries like React, Vue, or Angular leverage the DOM to efficiently render and manage components.
- Testing and automation: The DOM enables automated testing of web applications. Test frameworks can interact with the DOM, simulate user actions, and verify the expected behavior of the application, facilitating the development of robust and reliable web applications.
Drawbacks
- Performance overhead: The DOM can be resource-intensive, especially when dealing with large or complex web pages. Manipulating the DOM can lead to performance bottlenecks, as frequent updates or modifications to the DOM tree can result in slow rendering and responsiveness.
- Lack of native querying capabilities: The native DOM API lacks robust querying and traversal methods. Selecting elements based on complex criteria or navigating the DOM tree can be cumbersome and less efficient compared to modern JavaScript libraries and frameworks that provide enhanced querying capabilities, such as CSS selectors or XPath.
- Cross-browser inconsistencies: Although the DOM is a standardized specification, different web browsers may implement certain aspects of the DOM differently or have browser-specific quirks. This can lead to inconsistencies in behavior and appearance across different browsers, requiring additional effort and testing to ensure cross-browser compatibility.
- Security risks: Manipulating the DOM directly through JavaScript can introduce security vulnerabilities if not handled carefully. It opens the door to potential cross-site scripting (XSS) attacks.
- Inefficient batch updates: The DOM is designed for immediate updates and changes, and multiple small updates can trigger unnecessary reflows and repaints, negatively impacting performance. Techniques like batching DOM updates or using libraries/frameworks that optimize updates, like React’s virtual DOM, can help mitigate this issue.
- Performance limitations on mobile devices: Complex DOM manipulation and frequent updates can result in poor performance and increased battery drain on mobile devices.
React DOM
React DOM is responsible for rendering React components into the browser’s DOM, updating them efficiently, and keeping the user interface in sync with the underlying data. In other words, it is the specific implementation of the DOM for web applications built using the React javascript library. That means, React DOM is a package specifically designed for working with the DOM in React applications. It provides the necessary tools and functions to create, update, and manage the virtual representation of the UI components, and then efficiently render them into the real DOM.
The Virtual DOM in React optimizes UI updates by creating a lightweight representation of the actual DOM in memory, minimizing direct manipulations and improving performance.
When using React, instead of directly manipulating the DOM like you would with traditional JavaScript or jQuery, you define your user interface as a hierarchy of components using React’s JSX syntax. React DOM then takes care of efficiently updating the real DOM to reflect any changes in the component hierarchy.
Reconciliation/Diffing
Reconciliation is the technique used by React DOM, where it compares the previous and current version of the component hierarchy and makes only the necessary updates to bring the DOM in sync with the desired UI state which helps in optimizing performance and avoiding unnecessary re-renders of components.
React Virtual DOM
React DOM utilizes a concept called the virtual DOM. It creates a lightweight representation of the actual DOM in memory. This virtual DOM allows React to efficiently track and manage updates to the UI. When changes occur in the React components, React compares the previous and current virtual DOM representations and efficiently updates only the necessary parts of the actual DOM, minimizing performance overhead.
Component lifecycle methods
React DOM provides lifecycle methods that allow developers to define specific behavior during different phases of a component’s existence. These methods include initialization, updating, and unmounting stages. Developers can hook into these lifecycle methods to perform actions such as fetching data, setting up event listeners, or releasing resources.
Server-side rendering
React DOM supports server-side rendering (SSR), which enables rendering React components on the server and sending the pre-rendered HTML to the client. This helps improve performance and initial page load times, as the client receives already rendered content from the server, reducing the need for additional rendering on the client side.
Integration with the browser events
React DOM seamlessly integrates with browser events. It provides a way to attach event handlers to React components, allowing developers to respond to user interactions such as clicks, form submissions, or keyboard input. React’s event handling system normalizes browser-specific differences and provides a consistent interface for event handling.
Leave a comment
To make a comment, please send an e-mail using the button below. Your e-mail address won't be shared and will be deleted from our records after the comment is published. If you don't want your real name to be credited alongside your comment, please specify the name you would like to use. If you would like your name to link to a specific URL, please share that as well. Thank you.
Comment via email