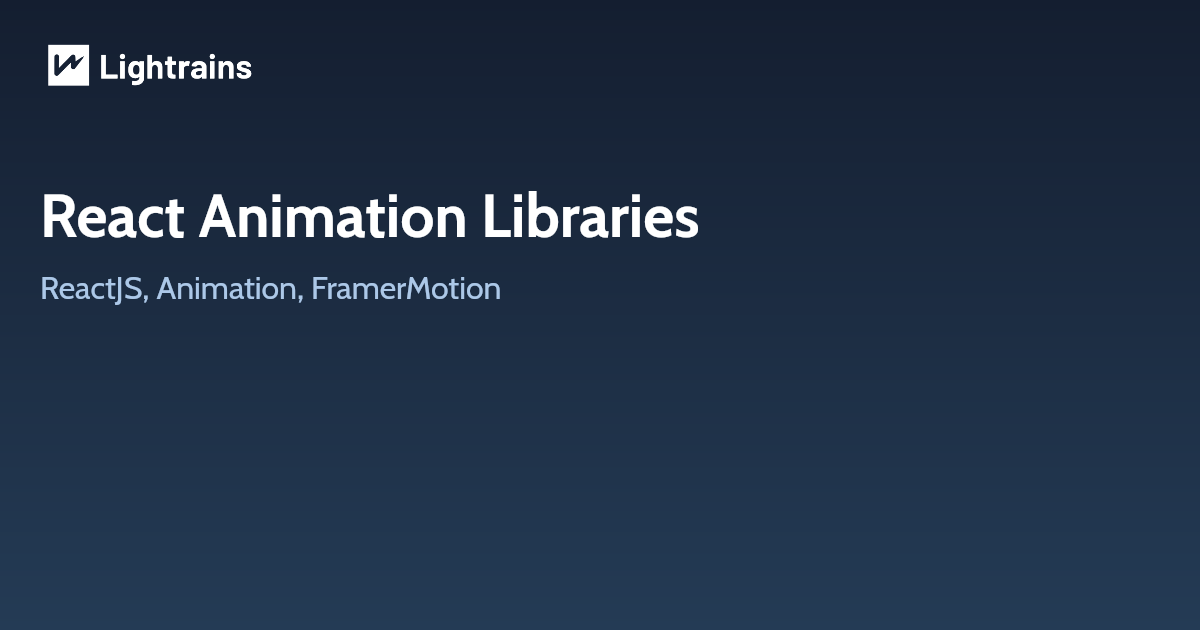
Animations are important in instances like page transitions, scroll events, entering and exiting components, and events that the user should be alerted to.
We’ll take a look at 3 React animation libraries that can be integrated with React for interactive and attractive web pages..
We will go over the following:
- Framer Motion
- React Anime
- React Spring
1. Framer Motion
Framer Motion is an open-sourced, production-ready React animation library that provides users with a non-complex API to easily create animations, manage animation properties, and handle user gestures such as clicks and drag. It has two APIs named Framer API and Motion API. Framer API is responsible for providing rapid interactions, while Motion API provides gestures and animations.
Features of Framer Motion
- Server-side rendering is well-supported by Framer Motion, making it compatible with React-based frameworks such as Next.js, which also offers server-side rendering capabilities.
- Colors are simple to adjust and manipulate.
- Helpers for complex, physics-based animation.
- Users only need to set the correct configurations. The Motion API automatically generates animations.
Installation of Framer Motion
You can easily install Framer Motion using NPM or Yarn as follows.
// NPM npm install framer-motion
// Yarn yarn add framer-motion
Usage of Framer Motion
Once you have installed it, you can incorporate Framer Motion into your React component as demonstrated below. The code snippet illustrates how to enlarge and reduce the size of a button when hovering over it.
import React from 'react'
import { motion } from 'framer-motion'
import './styles.css'
export default function App() {
return (
<div className="my-container">
<motion.div whileHover={{ scale: 1.2 }} whileTap={{ scale: 0.8 }} />
</div>
)
}
2. react-anime
Anime JS is a lightweight JavaScript animation library that offers a straightforward yet powerful API. It works with various elements such as CSS properties, SVG, DOM attributes, and JavaScript Objects.
Features of react-anime
- Easy to use. You can animate anything by wrapping it with the
<Anime>
component. - Ability to animate CSS, SVG, and DOM attributes just using props.
- Support for TypeScript.
Installation of react-anime
You can easily install react-anime using NPM and Yarn as follows.
npm i react-anime
yarn add react-anime
Usage of react-anime
After installation, you can use react-anime in your React application.
import React from 'react'
import Anime, { anime } from 'react-anime'
const App = props => (
<Anime
easing="easeOutElastic"
loop={true}
duration={1000}
direction="alternate"
delay={(el, index) => index * 240}
translateX="13rem"
scale={[0.75, 0.9]}>
<div className="blue" />
<div className="green" />
<div className="red" />
</Anime>
)
3. react-spring
react-spring is an advanced animation library that leverages the principles of physics, particularly the behavior of springs. It provides developers with remarkable flexibility and an array of impressive features, including hooks, designed to assist them in their animation tasks.
Features of react-spring
- For various scenarios, there are five hooks available in total: useChain, useSpring, useSprings, useTrail, and useTransition. Each of these hooks serves a specific purpose and can be used accordingly.
- Pre-defined configurations.
- Users can rapidly generate animations by supplying props to pre-defined components.
- Framer Motion offers cross-platform compatibility, supporting both web applications and native app development.
Installation of react-spring
You can easily install react-spring using NPM or Yarn as follows.
// NPM npm install react-spring
// Yarn yarn add react-spring
Usage of react-spring
Once you have installed it, you can import react-spring into your React component as demonstrated below. The code snippet showcases how to produce a fade-in animation.
import React from 'react'
import './style.css'
import { useSpring, animated } from '@react-spring/web'
export default function App() {
const styles = useSpring({
loop: true,
from: { opacity: '0' },
to: { opacity: '1' },
config: { duration: '2000' }
})
return (
<animated.div
style={{
width: 100,
height: 100,
backgroundColor: 'red',
borderRadius: 50,
...styles
}}
/>
)
}
This article originally appeared on lightrains.com
Leave a comment
To make a comment, please send an e-mail using the button below. Your e-mail address won't be shared and will be deleted from our records after the comment is published. If you don't want your real name to be credited alongside your comment, please specify the name you would like to use. If you would like your name to link to a specific URL, please share that as well. Thank you.
Comment via email