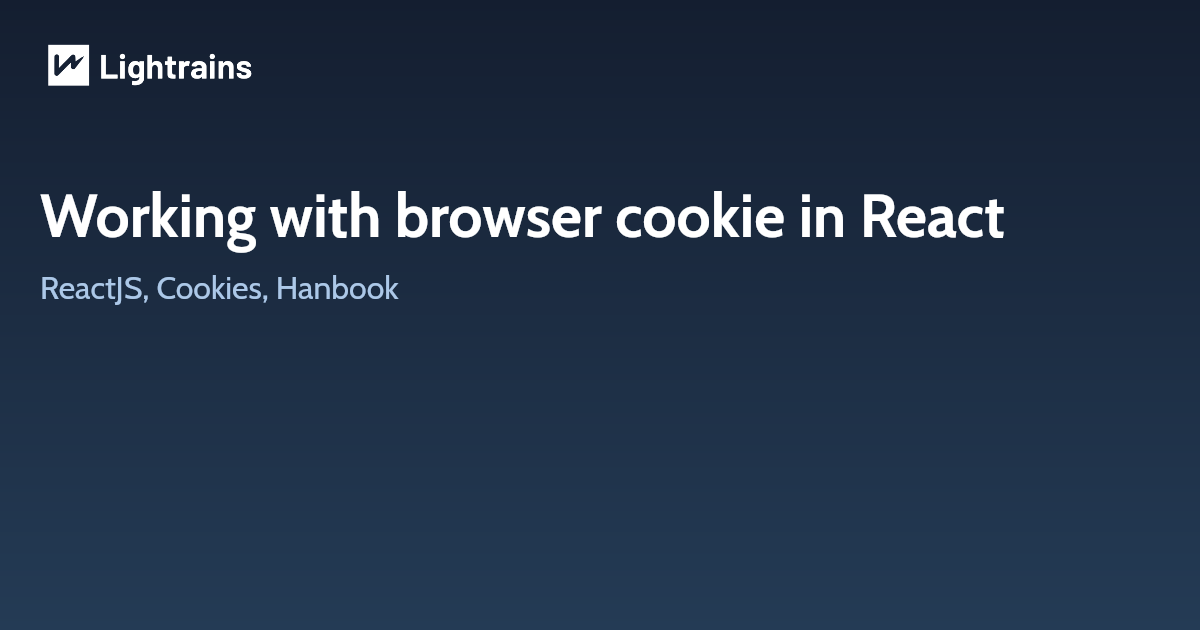
Cookies are an essential piece of component that is highly used when browsing any website in a web browser. Cookies are designed to be a reliable mechanism for websites to remember stateful information or to record the user’s browsing activity or verify the user identity. Overall, they optimize the internet as well as website experience for the end-users.
Installing the react-cookie package
First we need to install an (yarn) package called react-cookie in our project.
yarn add react-cookie
Setting Cookie
To start setting a cookie, first we will need to import CookieProvider
and wrap it around the main App component.
This is to be done in the index.js
file as shown in the code snippet below:
import React from 'react'
import ReactDOM from 'react-dom'
import { CookiesProvider } from 'react-cookie'
import App from './App'
const rootElement = document.getElementById('root')
ReactDOM.render(
<CookiesProvider>
<App />
</CookiesProvider>,
rootElement
)
App.jsx
import React, { useState } from 'react'
import { useCookies } from 'react-cookie'
const App = () => {
const [name, setName] = useState('')
const [pwd, setPwd] = useState('')
const [cookies, setCookie] = useCookies(['user'])
const handle = () => {
setCookie('Name', name, { path: '/' })
setCookie('Password', pwd, { path: '/' })
}
return (
<div className="App">
<h1>Name</h1>
<input
placeholder="name"
value={name}
onChange={e => setName(e.target.value)}
/>
<h1>Password</h1>
<input
type="password"
placeholder="name"
value={pwd}
onChange={e => setPwd(e.target.value)}
/>
<div>
<button onClick={handle}>Set Cookie</button>
</div>
</div>
)
}
export default App
Checkout example CodeSandbox
Conclusion
With react-cookie plugin, react developers are having easier time to work with the cookies in a react-like environment instead of going back to the default javascript method.
This article originally appeared on lightrains.com
Leave a comment
To make a comment, please send an e-mail using the button below. Your e-mail address won't be shared and will be deleted from our records after the comment is published. If you don't want your real name to be credited alongside your comment, please specify the name you would like to use. If you would like your name to link to a specific URL, please share that as well. Thank you.
Comment via email