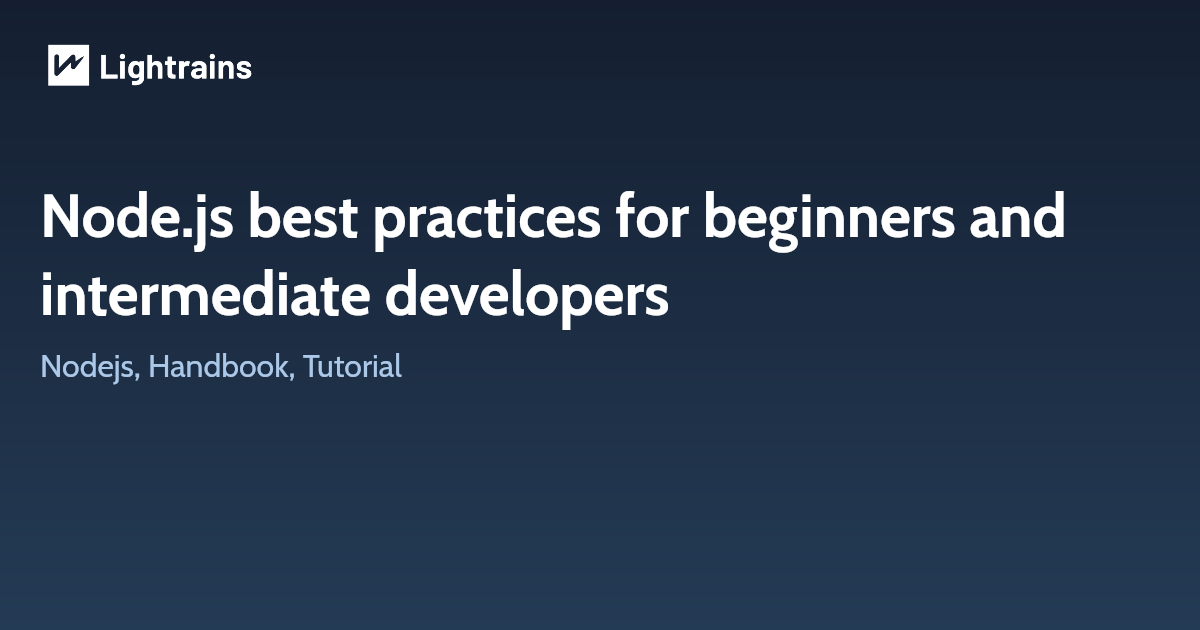
Node.js is an open-source, cross-platform, back-end JavaScript runtime environment that runs on the V8 engine and executes JavaScript code outside a web browser.
Node.js has an event-driven architecture capable of asynchronous I/O. These design choices aim to optimize throughput and scalability in web applications with many input/output operations, as well as for real-time Web applications.
Since the Initial release May 27, 2009 (12 years); node.js has been one of the favorite language of developers across the globe. According to SO 2021 Developer survey Node.js is the goto programming language for 36.19% of total developers.
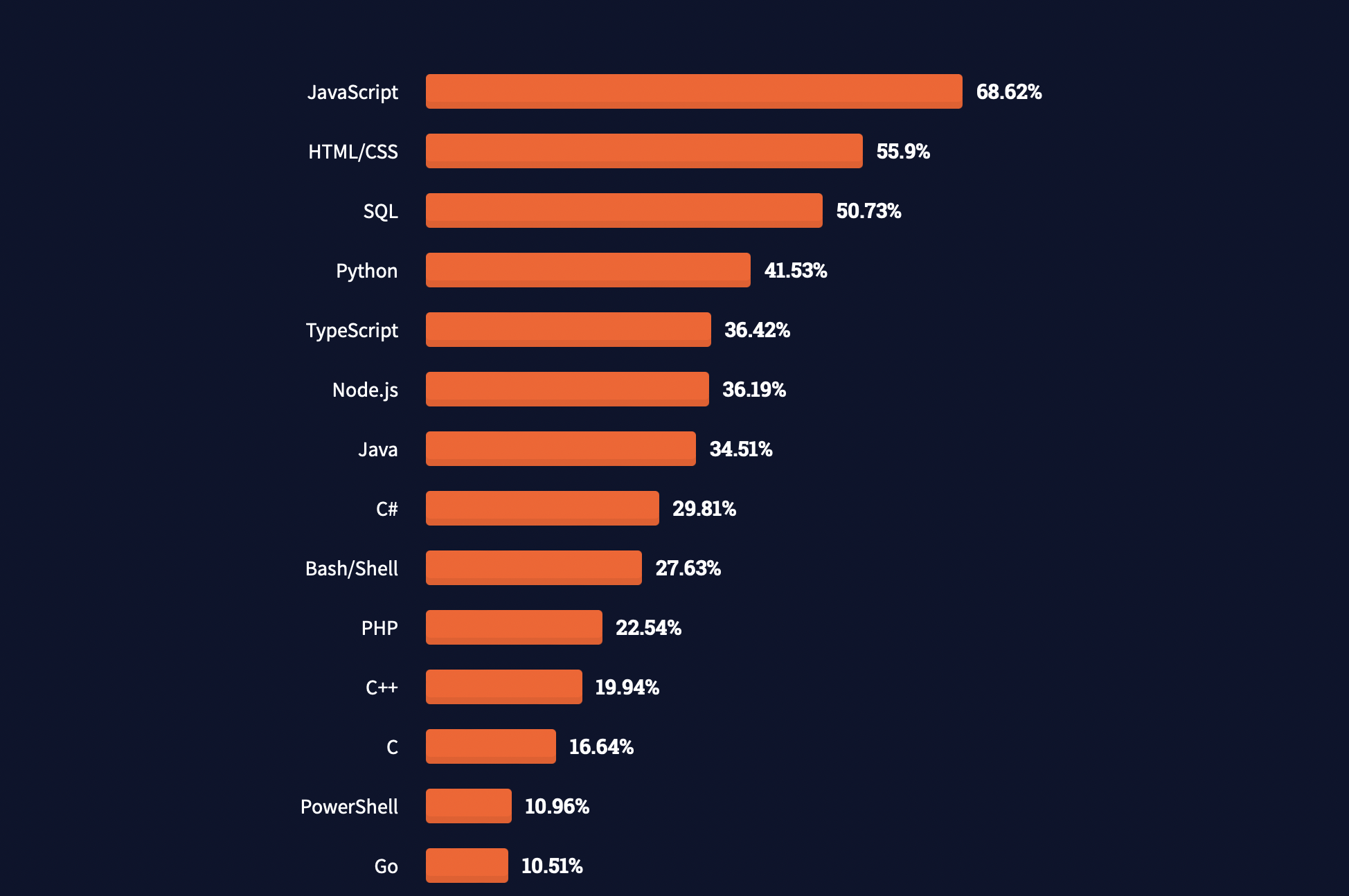
We Lightrains use Node.js as our goto stack for rapid prototyping and even in applications which require high tps and flexibility.
Following are our main strategies when we approach any project where the main stack is Node.js
1. Start a project with stable modules.
Always try to create a new project with your package manager npm
, yarn
or pnpm
; we use yarn
and yarn is used extensively in our CI scripts and other automation tools.
mkdir new-project && cd "$_"
yarn init -y
Follow the onscreen prompt and give necessary details.
This will create a file names package.json
with following contents
{
"name": "new-project",
"version": "1.0.0",
"main": "index.js",
"license": "MIT"
}
2. Add scripts to your application
Scripts are a great way of automating tasks related to your package, such as simple build processes or development tools.
There is a standard way to start a node application add start
script properly using script
tag so that it can be started an example would look like the following
...
"scripts" : {
"start": "node ./src/app.js"
}
...
When someone runs npm/yarn/pnpm start
the app would run node ./src/app.js
3. Use a proper style guide
There are many famous style guide available in the industry since it is ao big, many companies and communities built their own style guide and made them open source a few famous ones are
An interesting article about Coding Style
Also consider enforcing tools to enforce these standard. A few common tools are Prettier, ESLint and Husky
4. Use Environment variables instead of hard-coding
Use environment variables instead of hard-coding them in the code. The process core module of Node.js provides the env property which hosts all the environment variables that were set at the moment the process was started.
The below code runs app.js and set USER_ID and USER_KEY.
USER_ID=9895 USER_KEY=admin_user node app.js
Here is an example that accesses the USER_ID and USER_KEY environment variables, which we set in above code.
process.env.USER_ID // "239482"
process.env.USER_KEY // "foobar"
If you have multiple environment variables in your node project, you can also create an .env file in the root directory of your project, and then use the dotenv package to load them during runtime.
# .env file
USER_ID="9895"
USER_KEY="admin_user"
NODE_ENV="development"
In your js file
require('dotenv').config()
process.env.USER_ID // "9895"
process.env.USER_KEY // "admin_user"
process.env.NODE_ENV // "development"
How to read environment variables from Node.js
5. Make use of Asyc api
The synchronous function makes the flow of application logic easy to understand. However, it blocks any other code from running until it gets complete. You can trace the synchronous function in the code using the -trace-sync-io flag, it will display a warning when encountered with the synchronous API.
6. Handle Errors Properly
Assuming you have some experience with async JavaScript and Node.js, you might have experienced drawbacks when using callbacks for dealing with errors. They force you to check errors all the way down to nested ones, causing notorious “callback hell” issues that make it hard to follow the code flow.
Using promises or async/await is a good replacement for callbacks. The typical code flow of async/await looks like the following:
const anAsyncTask = async () => {
try {
const user = await getUser()
const cart = await getCart(user)
return cart
} catch (error) {
console.error(error)
} finally {
await cleanUp()
}
}
You can use the built-in Error object in Node.js as I mentioned above, as it gives you detailed info about stack traces. However, I also want to show you how to create custom Error objects with more meaningful properties like HTTP status codes and more detailed descriptions.
Here’s a file called baseError.js
where you set the base for every custom error you’ll use.
// baseError.js
class BaseError extends Error {
constructor(name, statusCode, isOperational, description) {
super(description)
Object.setPrototypeOf(this, new.target.prototype)
this.name = name
this.statusCode = statusCode
this.isOperational = isOperational
Error.captureStackTrace(this)
}
}
module.exports = BaseError
The strategy of handling errors in a single component in Node.js will ensure developers save valuable time and write clean and maintainable code by avoiding code duplication and missing error context.
7. Use logging tools
Console.log is a great tool but has its limitations in a production application therefore you can not use it for every logging purpose. It does not provide enough configuration options, for example, there is no filter option to filter loggings. Some good examples of logging frameworks are Bunyan, Winston, and Pino. It makes Node.js logging simpler and more efficient.
8. Test your application
It is crucial to test your application before launching it in the market. No matter what stage of your application development, it is never too late to introduce testing. Experts recommend writing a test for every bug that gets reported.
Therefore you must know:
- How to recreate the bug (make sure your test fails first!)
- How to fix the bug (make sure you pass the test once the bug is fixed)
- Make sure that the bug will never occur again. Following are the most popular testing Libraries for Node.is applications
This article originally appeared on lightrains.com
Leave a comment
To make a comment, please send an e-mail using the button below. Your e-mail address won't be shared and will be deleted from our records after the comment is published. If you don't want your real name to be credited alongside your comment, please specify the name you would like to use. If you would like your name to link to a specific URL, please share that as well. Thank you.
Comment via email