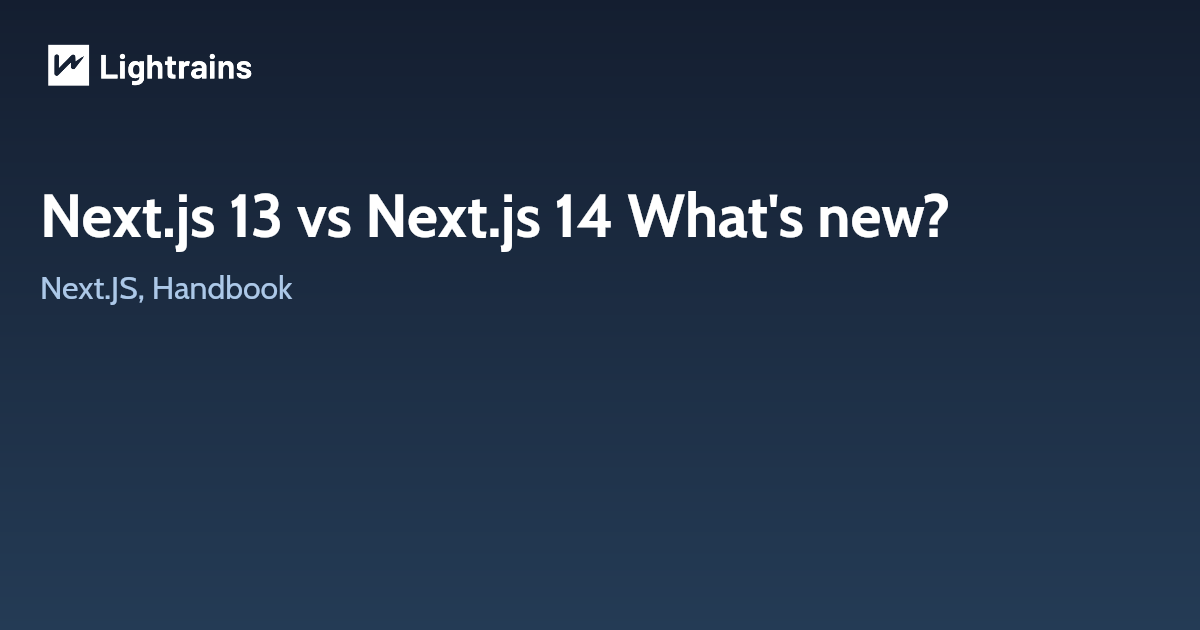
Next.js is a popular React.js framework for building complex and performant web applications. Since its launch in 2016, Next.js has been constantly improved and updated for better performance and a great developer experience.
Next.js 13 and Next.js 14 are two major versions of the Next.js framework. Next.js 13 was released in October 2022, and Next.js 14 was released in October 27, 2023.
Both versions introduce a number of new features and improvements, but Next.js 14 has the added advantage of supporting React 19 features. The main difference between Next.js 13 and Next.js 14 is the addition of Server Actions and Partial Prerendering.
Server Actions: Server Actions are asynchronous functions that can be used to perform server-side operations, such as fetching data from a database or sending an email. They are similar to React Actions, but they are executed on the server instead of the client.
Partial Prerendering: Partial Prerendering allows you to render dynamic content on the server, even if it depends on user input. This can improve the performance of your application, especially for initial page loads.
Other notable differences
Other notable differences between Next.js 13 and Next.js 14 include:
- TurboPack: TurboPack is a new Rust-powered build engine that is significantly faster than the previous build engine.
- Support for React Native: Next.js 14 now supports React Native, allowing you to build hybrid web and mobile applications with the same codebase.
- New image optimization features: Next.js 14 includes a number of new image optimization features, such as automatic image resizing and support for WebP images.
- Improved SEO support: Next.js 14 includes a number of improvements to SEO support, such as the ability to generate sitemaps and dynamic canonical URLs.
New features in Next.js 13
- App directory: A new app directory that provides a more flexible and scalable way to structure your application code.
- Server components: Allow you to render React components on the server without having to write any boilerplate code.
- React 18 support: Support for React 18 features such as automatic batching and streaming.
- Performance improvements: A number of performance improvements, including a new bundle optimizer and a faster development server.
New features in Next.js 14
- React 19 support: Support for React 19 features such as concurrent rendering and the useTransition hook.
- Built-in image optimizer: A new built-in image optimizer that can significantly reduce the size of your application’s image assets.
- Next.js CLI: A new Next.js CLI that makes it easier to manage your Next.js projects.
- Performance improvements: A number of performance improvements, including a new incremental static regeneration (ISR) algorithm and a faster dev server.
New features and improvements in Next.js 14 with code snippets
Here are some of the new features and improvements in Next.js 14 with code snippets:
1. Support for React 19 features
Concurrent rendering
Concurrent rendering has been a major feature in React 18, and it is now supported in Next.js 14. Concurrent rendering allows React to render multiple versions of a component at the same time. This can improve the performance of your application by allowing React to render updates to the UI without blocking the main thread.
useTransition hook
The useTransition
hook is another new feature in React 19. This hook allows you to transition between states in a controlled way. It can be used to implement loading states, animations, and other effects.
Example:
// pages/index.jsx
import { useState, useTransition } from 'react'
const IndexPage = () => {
const [isLoading, setIsLoading] = useState(true)
const [data, setData] = useState([])
const transition = useTransition()
async function fetchData() {
const response = await fetch('https://api.example.com/data')
const data = await response.json()
setData(data)
setIsLoading(false)
}
useEffect(() => {
fetchData()
}, [])
if (transition.isRunning || isLoading) {
return <div>Loading...</div>
}
return (
<ul>
{data.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
)
}
export default IndexPage
2. Built-in image optimizer
Next.js 14 includes a built-in image optimizer that can significantly reduce the size of your application’s image assets. This can lead to faster page load times and improved user experience.
Example:
// pages/index.jsx
import { Image } from 'next/image'
const IndexPage = () => {
return (
<div>
<Image
src="/image.png"
alt="My image"
width={500}
height={500}
quality={75}
/>
</div>
)
}
export default IndexPage
The quality
prop in the above example tells Next.js to compress the image by 25%. This will reduce the size of the image without sacrificing too much quality.
3. Next.js CLI
Next.js 14 includes a new CLI tool that makes it easier to manage your Next.js projects. The CLI provides a number of commands for tasks such as creating new projects, starting the development server, and building and deploying your application.
Example:
# Create a new Next.js project
yarn create next-app my-project
# Start the development server
yarn dev
# Build the application for production
yarn build
# Deploy the application to Vercel
yarn deploy
4. Performance improvements
Next.js 14 includes a number of performance improvements, such as a new incremental static regeneration (ISR) algorithm and a faster dev server.
Incremental static regeneration (ISR) ISR allows Next.js to generate static pages for your application at build time. This can improve the performance of your application by reducing the number of requests that need to be made to the server.
Faster dev server The new dev server in Next.js 14 is faster than the old dev server. This means that your application will start up and reload more quickly.
Overall, Next.js 14 is a significant release of the popular Next.js framework. It includes a number of new features and improvements that can help you to build better performing and more efficient Next.js applications.
Next.js 14 includes a number of improvements to metadata handling, including:
- Decoupling of blocking and non-blocking metadata: In previous versions of Next.js, all metadata was blocking, meaning that it had to be loaded before the page could start rendering. In Next.js 14, only a small subset of metadata is blocking, which can improve the perceived performance of your application.
- Support for file-based metadata: In addition to the traditional inline metadata, Next.js 14 now supports file-based metadata. This allows you to define your metadata in a separate file, which can be useful for managing large or complex metadata.
- Improved metadata validation: Next.js 14 includes improved metadata validation, which can help you to catch errors early in the development process. Here is a code snippet showing how to use file-based metadata in Next.js 14:
JavaScript
// metadata.json
{
"title": "My Next.js Application",
"description": "This is my Next.js application."
}
// index.js
import metadata from './metadata.json';
function IndexPage() {
return (
<div>
<h1>{metadata.title}</h1>
<p>{metadata.description}</p>
</div>
);
}
export default IndexPage;
In Next.js 13, the above code would not work, as file-based metadata was not supported.
Overall, the metadata handling in Next.js 14 is more flexible and powerful than in Next.js 13. It is now possible to define non-blocking metadata, use file-based metadata, and benefit from improved metadata validation.
Conclusion
Next.js 13 and Next.js 14 are both excellent versions of the Next.js framework. They both introduce a number of new features and improvements, but Next.js 14 has the added advantage of supporting React 19 features.
This article originally appeared on lightrains.com
Leave a comment
To make a comment, please send an e-mail using the button below. Your e-mail address won't be shared and will be deleted from our records after the comment is published. If you don't want your real name to be credited alongside your comment, please specify the name you would like to use. If you would like your name to link to a specific URL, please share that as well. Thank you.
Comment via email