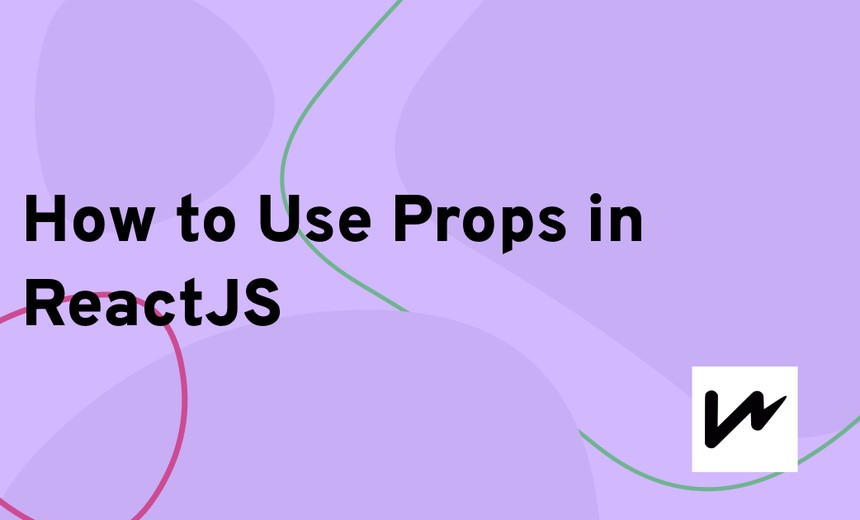
What are props?
Props is short for properties. React has a special object called a prop which we use to transport data from one component to another.
Props only transport data in a one-way flow (only from parent to child components). It is not possible with props to pass data from child to parent, or to components at the same level.
component App.js
looks like:
function MyApp() {
return <div className="App"></div>
}
export default MyApp
Now let’s create another component named Welcome.jsx
export default function Welcome= () => {
return (
<div>
<h1>Hello</h1>
</div>
);
}
Next we will now import this component into the App
component. That is:
import Welcome from './Welcome'
function MyApp() {
return (
<div className="App">
<Welcome />
</div>
)
}
export default MyApp
We know that components in React are used again and again in the UI, but we don’t normally render the same component with the same data. Sometimes we need to change the content inside a component. Props come to play in these cases, as they are passed into the component.
First, we need to define a prop on the Welcome Component and assign a value to it:
import Welcome from './Welcome'
function MyApp() {
return (
<div className="App">
<Welcome name="John" />
<Welcome name="Mary" />
<Welcome name="Alex" />
</div>
)
}
export default MyApp
Then we can pass the values and get the result simply by accessing the prop “name”:
export default function Welcome= ({ props }) => {
return (
<div>
<h1>Hello {props.name} </h1>
</div>
);
}
Output :
Hello John
Hello Mary
Hello Alex
Passing a state as a prop
import { useState } from "react"
import Welcome from './Welcome';
export default function MyApp= () => {
const [list, setList] = useState("Hello")
return (
<div>
<Welcome list={list}/>
</div>
);
}
How to set default values for props
import { useState } from "react"
import Welcome from './Welcome';
export default function MyApp= () => {
const [list, setList] = useState(
[
{
name: "John",
age: 14,
},
{
name: "Mary",
age: 13,
}
]);
return (
<div>
<Welcome list={list}/>
</div>
);
}
How to send state/props to another component in React with onClick?
- App.js:
import React, { useState } from 'react'
import Component from './Component'
function App() {
const [state, setstate] = useState({ data: '' })
const changeState = () => {
setstate({
data: `state/props of parent component
is send by onClick event to another component`
})
}
return (
<div className="App">
<Component data={state.data} />
<div>
<h2>Compnent1</h2>
<button onClick={changeState} type="button">
Submit
</button>
</div>
</div>
)
}
export default App
2.Component.jsx:
import React from 'react'
export default function Component(props) {
return (
<div>
<h2>Compnent</h2>
<p>{props.data} </p>
</div>
)
}
Differences between props and state
PROPS
- The Data is passed from one component to another.
- It is Immutable (cannot be modified).
- Props can be used with state and functional components.
- Props are read-only.
STATE
- The Data is passed within the component only.
- It is Mutable ( can be modified).
- State can be used only with the state components.
- State is both read and write.
Leave a comment
To make a comment, please send an e-mail using the button below. Your e-mail address won't be shared and will be deleted from our records after the comment is published. If you don't want your real name to be credited alongside your comment, please specify the name you would like to use. If you would like your name to link to a specific URL, please share that as well. Thank you.
Comment via email