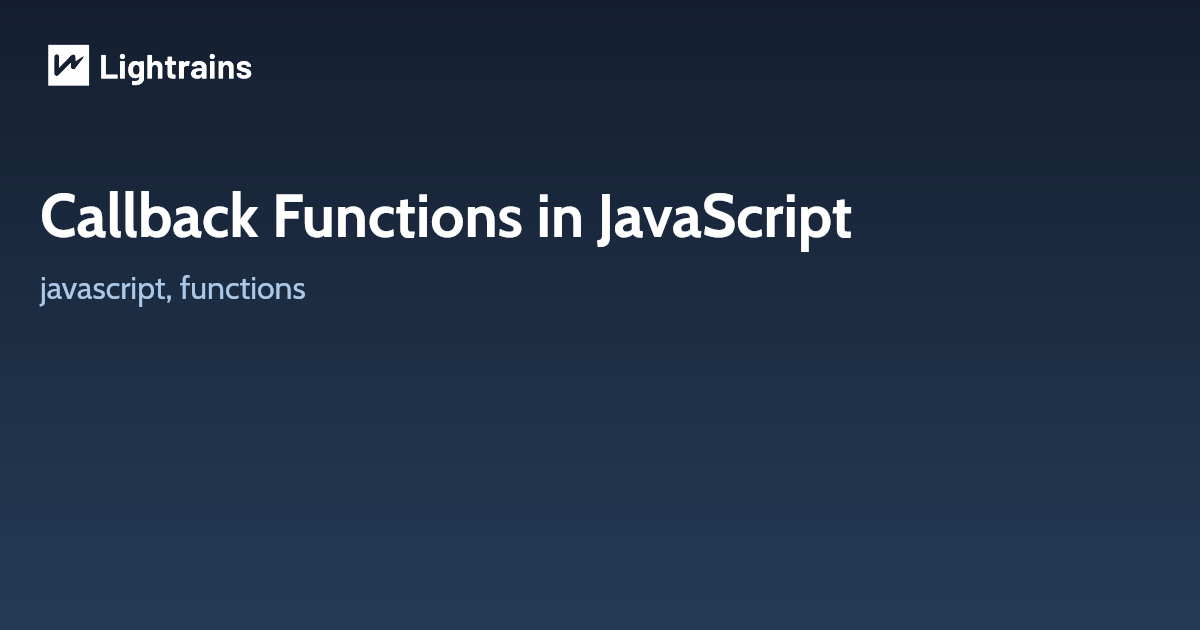
While writing a program for a small scale application to an enterprise-level application function plays an important role. The functions keep the program organized, easy to understand and makes it reusable and increase the readability of a program. In this tutorial, we are discussing callback function with an example.
Callback Functions
The callback function is a function which is passed as an argument to another function. A callback function executes within the function to which it is passed. Callback functions are also known as high order functions. mdn
Writing a Callback Function
let functionOne = function () {
console.log(' I am a function , I am called inside a function.')
}
let functionTwo = function (callback) {
console.log('I have a callback function.')
callback()
}
functionTwo(functionOne)
Let’s dive into the above example. We have defined two functions functionOne and functionTwo. functionOne
is a function without any parameter whereas functionTwo
accepts a callback as a parameter. When functionTwo
is called we pass functionOne
as an argument. The output of above code is as follows.
I have a callback function.
I am a function, I am called inside a function.
functionOne
will only execute after functionTwo complete its execution. Thus functionTwo
is a callback function which accepts a function as a parameter. Callback helps in asynchronous programming, simply means execute something after something else has executed. Consider the below example. The setTimeout()
method will execute a function after a specific time.
setTimeout(function() => {
console.log(‘Hello’);
}, 5000);
The above code prints the string Hello in the terminal after five seconds.
Consider this example creating a new file in NodeJS.
Syntax
fs.writeFile(file, data, options, callback)
const fs = require('fs')
fs.writeFile('file.txt', 'Hello world', function (err) {
if (err) {
throw err
} else {
console.log('Done')
}
})
The fs.writeFile()
method replaces the file and content in ‘file.txt’ if it exists. If the ‘file.txt’ file does not exist, a new file, containing the content ‘Hello world’, will be created. Callback is an error that would be thrown if the file operation fails. otherwise print a message ‘Done’ to the console.
When to Use a Callback?
The examples above are not very exciting. They are simplified to teach you the callback syntax. Where callbacks really shine are in asynchronous functions, where one function has to wait for another function (like waiting for a file to load).
This article aims to provide an introduction to callback functions for the beginners in JavaScript. I hope the concept of the callback is clear. Thanks for reading.
This article originally appeared on lightrains.com
Leave a comment
To make a comment, please send an e-mail using the button below. Your e-mail address won't be shared and will be deleted from our records after the comment is published. If you don't want your real name to be credited alongside your comment, please specify the name you would like to use. If you would like your name to link to a specific URL, please share that as well. Thank you.
Comment via email